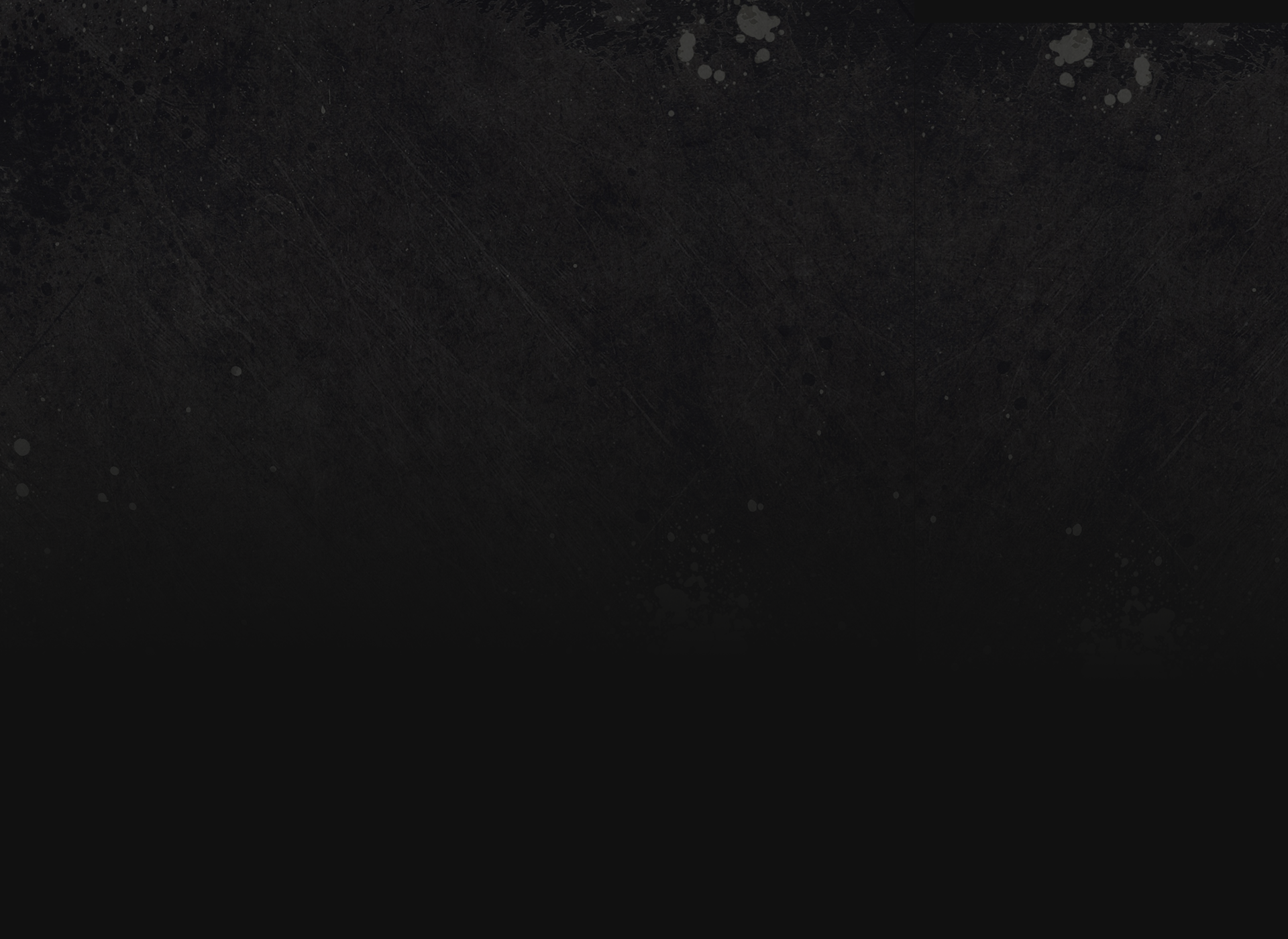
MAKE REVIVE SCRIPT V.1
Thanks to Babast from FOXCOM TEAM for this script
To make respawn system like in wasteland with revive, stabilize and drag option, follow theses steps :
​
STEP 1 : Make INIT.sqf file inside your mission folder and past this inside :
​
​
/* Compile basstard's revive */
call compileFinal preprocessFile "addons\BAS_revive\BAS_revive_initialization.sqf";
​
​
STEP 2 : Make onPlayerRespawn.sqf file inside your mission folder and past this inside :
​
​
/******************************************************************************************
* This work is licensed under the APL-SA. Copyright © 2016 basstard @ BI Forums *
******************************************************************************************
Author : basstard
Date : 19/08/2016 (jj/mm/aaaa)
Version : 0.1
Description : Player initialization script on respawn
Usage : This script is an event script called by the engine.
Syntax : N/A
Parameters : [player:Object, didJIP:Boolean]
*/
private _corpse = _this select 1;
if (!isNull _corpse) then
{
/* Add action to hide body on player's corpse */
_corpse remoteExec ["BAS_revive_fnc_addHideBodyAction", [0,-2] select isDedicated, _corpse];
};
[player, [missionNamespace, "inventory_var"]] call BIS_fnc_loadInventory;
/* Initialize player for basstard's revive functionnalities */
[] spawn BAS_revive_fnc_playerInit;
​
​
STEP 3 : Make addons folder inside your mission folder, and make BAS_revive folder inside addons folder
​
STEP 4 : inside BAS_revive folder, make BAS_revive_functions.sqf file and past inside :
​
​
/******************************************************************************************
* This work is licensed under the APL-SA. Copyright © 2016 basstard @ BI Forums *
******************************************************************************************
Author : basstard
Date : 19/08/2016 (jj/mm/aaaa)
Version : 0.1
Description : Functions collection for basstard's revive functionnalities
Usage : N/A
Syntax : N/A
Parameters : None
*/
#include "BAS_revive_macros.sqf"
/******************************************************************************************
Description : Initialize player object
Syntax : [] call BAS_revive_fnc_playerInit;
Parameters : None
Return : None
*******************************************************************************************/
BAS_revive_fnc_playerInit =
{
/* Wait if player is JIP */
waitUntil { !isNull player };
/* Avoid multiple player init */
if (player getVariable ["BAS_revive_initDone", false]) exitWith {};
/* Cache player object */
private _myPlayerObject = player;
/* Disable fatigue system */
_myPlayerObject enableFatigue false;
/* Disable captive status */
_myPlayerObject setCaptive false;
/* Remove all existing Event Handlers */
waitUntil {!isNil {_myPlayerObject getVariable "BIS_fnc_feedback_hitArrayHandler"}};
_myPlayerObject removeAllEventHandlers "HandleDamage";
/* Add EHs to player */
_myPlayerObject addEventHandler ["HandleDamage", BAS_revive_fnc_handleDamage];
_myPlayerObject addEventHandler ["Killed", BAS_revive_fnc_handleDeath];
/* Intialize player variables */
_myPlayerObject setVariable ["BAS_isMedic", getNumber (configfile >> "CfgVehicles" >> typeOf _myPlayerObject >> "attendant") isEqualTo 1, true];
_myPlayerObject setVariable ["BAS_isEngineer", getNumber (configfile >> "CfgVehicles" >> typeOf _myPlayerObject >> "engineer") isEqualTo 1, true];
_myPlayerObject setVariable ["BAS_isIncapacitated", false, true];
_myPlayerObject setVariable ["BAS_isRevived", false, true];
_myPlayerObject setVariable ["BAS_isStabilized", false, true];
_myPlayerObject setVariable ["BAS_isDragged", false, true];
_myPlayerObject setVariable ["BAS_animDone", "", true];
_myPlayerObject setVariable ["BAS_playerSide", side _myPlayerObject, true]; // cache side because when set captive a unit become civilian
/* Disable speech and radio messages */
_myPlayerObject setVariable ["BIS_noCoreConversations", true];
0 fadeRadio 0;
enableSentences false;
/* Add action to allow player to empty his hands (runs faster) */
_myPlayerObject addAction ["Empty Hands", { (_this select 0) action ["SwitchWeapon", _this select 0, _this select 0, 100]}, nil, 5, false, true, "", '((currentWeapon _target) != "") && (isNull objectParent player)', -1, false];
#ifdef DEBUG
_myPlayerObject addAction ["<t color='#FF0000'>Damage player</t>", {(_this select 0) setDamage 0.76}, nil, 10, false, true, "", "true", -1, false];
#endif
/* Acknowledge initialization */
_myPlayerObject setVariable ["BAS_revive_initDone", true, false];
};
/******************************************************************************************
Description : Handle damages to player (called from EH)
Syntax : N/A
Parameters : None
Return : Number
*******************************************************************************************/
BAS_revive_fnc_handleDamage =
{
private ["_unit", "_hitPart", "_partDamage", "_actualDamage", "_projectile", "_isIncapacitated", "_isCritical", "_isLethal"];
_unit = _this select 0;
_isIncapacitated = _unit getVariable "BAS_isIncapacitated";
if (_isIncapacitated) then
{
_partDamage = 0; // negate damage when unit is incapacitated
}
else
{
_hitPart = _this select 1;
_partDamage = _this select 2;
_projectile = _this select 4;
_isCritical = _hitPart in ["","head","face_hub","neck","body","spine1","spine2","spine3","pelvis"]; // ignore shots to arms, hands and legs
_actualDamage = if (_hitPart == "") then { _partDamage = _partDamage * OVERALL_DAMAGE_MODIFIER; damage _unit } else { _unit getHit _hitPart }; // use damage modifier to lower overall damage and focus on body part damage
if ((alive _unit) && ((_actualDamage + _partDamage) > 1) && (_isCritical)) then
{
/* Handle incapacitated or dead */
if ((!(_hitPart in ["head","face_hub","neck"])) && (_projectile call BAS_revive_fnc_isProjectileNonLethal)) then // because you can't be revived from headshots, explosives and high caliber rounds
{
/* Set incapacitated status */
_unit setVariable ["BAS_isIncapacitated", true, true];
/* Negate the damage received from this shot */
_partDamage = 0; // player won't eventually get killed when the EH returns
/* Handle incapacitated status */
_unit spawn BAS_revive_fnc_playerIncapacitated;
}
else
{
_unit setDamage 1;
};
};
};
_partDamage // this value will overwrite the default damage of given selection after processing
};
/******************************************************************************************
Description : Make the player incapacitated (can't move and bleeds until death or being revived or stabilized)
Syntax : [] spawn BAS_revive_fnc_playerIncapacitated;
Parameters : None
Return : None
*******************************************************************************************/
BAS_revive_fnc_playerIncapacitated =
{
private ["_unit", "_bleedOut", "_EHanimDoneID", "_progressBar", "_progressBarText", "_remainingBlood"];
_unit = _this;
disableUserInput true;
_unit allowDamage false;
_unit setDamage 0;
_unit setCaptive true;
/* Test if player was attached to an object (e.g. dragging someone) */
{
if (_x isKindOf "CAManBase") exitWith { detach _x }
} count attachedObjects _unit;
/* Test if player was in a vehicle when he was incapacitated */
if (!isNull objectParent _unit) then
{
/* Eject player from vehicle */
unAssignVehicle _unit;
_unit action ["getOut", objectParent _unit];
/* Wait until player hits the ground */
// waitUntil { sleep 0.5; isTouchingGround _unit };
waitUntil { sleep 0.5; isNull objectParent _unit };
};
/* Immobilize player */
_unit setVelocity [0,0,0]; // workaround to avoid weird behavior, like player being on is back and still slightly moving
/* Play "death" animation */
SET_ANIM(_unit);
_EHanimDoneID = _unit addEventHandler ["AnimDone", BAS_revive_fnc_handleAnim];
if ((stance _unit) in ["STAND","CROUCH"]) then
{
[_unit, "AcinPercMrunSrasWrflDf_agony"] call BAS_revive_fnc_switchMoveGlobal;
}
else
{
_unit playMove "AinjPpneMstpSnonWrflDnon_rolltoback";
};
waitUntil { GET_ANIM(_unit) != "" };
_unit removeEventHandler ["AnimDone", _EHanimDoneID];
SET_ANIM(_unit);
/* Force camera view to 1st person */
if (cameraView != "INTERNAL") then
{
_unit switchCamera "INTERNAL";
};
_unit setUnconscious true;
/* Add actions to incapacitated player object on all clients */
_unit remoteExec ["BAS_revive_fnc_addReviveActions", [0,-2] select isDedicated, _unit];
if (BAS_revive_allowPlayerExecution) then
{
_unit remoteExec ["BAS_revive_fnc_addExecutionAction", [0,-2] select isDedicated, _unit];
};
/* Display dialog with progress bar and suicide button */
disableUserInput false;
/* Initialize progress bar */
// _progressBar progressSetPosition 1;
// _progressBarText ctrlSetText "Bleeding";
/* Wait for revive or death loop */
_bleedOut = diag_tickTime + BAS_revive_bleedOut;
while { (alive _unit) && (_unit getVariable "BAS_isIncapacitated") && (diag_tickTime < _bleedOut) } do
{
/* Handle progress Bar */
if (_unit getVariable "BAS_isStabilized") then
{
// _progressBar progressSetPosition 1;
// _progressBarText ctrlSetText "Stabilized";
_bleedOut = diag_tickTime + BAS_revive_bleedOut; // when stabilized, this allows to never bleed out to death
hint "You are stabilized";
}
else
{
_remainingBlood = (_bleedOut - diag_tickTime) / BAS_revive_bleedOut;
// _progressBar progressSetPosition _remainingBlood;
hint format ["Blood: %1", round (_remainingBlood * 100)];
/* Add blood effect to player's screen */
[10 + floor ((1 - _remainingBlood) * 100)] call BIS_fnc_bloodEffect; // increase blood effect with time elapsing
};
uiSleep 1;
};
/* Remove hint for blood */
hint "";
/* Handle revived or dead state */
if (alive _unit) then
{
if (_unit getVariable "BAS_isIncapacitated") then
{
/* Player bled out*/
_unit setCaptive false;
_unit allowDamage true;
_unit setDamage 1;
}
else
{
/* Player got revived */
/* Play the animation corresponding to the weapon he was holding before being incapacitated */
switch (currentWeapon _unit) do
{
case (primaryWeapon _unit): { _unit playMove "AmovPpneMstpSrasWrflDnon" };
case (secondaryWeapon _unit): { _unit playMove "AmovPpneMstpSrasWlnrDnon" };
case (handgunWeapon _unit): { _unit playMove "AmovPpneMstpSrasWpstDnon" };
default { _unit playMove "AmovPpneMstpSrasWnonDnon" };
};
/* Remove incapacitated status */
_unit allowDamage true;
_unit setCaptive false;
_unit setUnconscious false;
_unit setVariable ["BAS_isStabilized", false, true];
};
};
/* Close dialog */
// disableUserInput false;
/* Remove add revive actions from JIP queue */
remoteExec ["", _unit];
/* Remove revive actions from incapacitated player object on all clients */
_unit remoteExec ["removeAllActions", [0,-2] select isDedicated, false];
};
/******************************************************************************************
Description : Handle AnimDone event handler
Syntax : [] call BAS_revive_fnc_handleAnim;
Parameters : None
Return : None
*******************************************************************************************/
BAS_revive_fnc_handleAnim =
{
private _unit = _this select 0;
private _anim = _this select 1;
switch (_anim) do
{
case "ainjppnemstpsnonwrfldnon": { _unit setVariable ["BAS_animDone", _anim, true] };
case "ainjppnemstpsnonwrfldb_release": { _unit setVariable ["BAS_animDone", _anim, true] };
case "ainvpknlmstpslaywrfldnon_medicother": { _unit setVariable ["BAS_animDone", _anim, true] };
case "ainvppnemstpslaywrfldnon_medicother": { _unit setVariable ["BAS_animDone", _anim, true] };
case "acinpercmrunsraswrfldf_agony": { _unit setVariable ["BAS_animDone", _anim, true] };
case "ainjppnemstpsnonwrfldnon_rolltoback": { _unit setVariable ["BAS_animDone", _anim, true] };
case "acts_executioner_kill": { _unit setVariable ["BAS_animDone", _anim, true] };
case "unconsciousrevivedefault": { _unit setVariable ["BAS_animDone", _anim, true] };
default { _unit setVariable ["BAS_animDone", "", true] };
};
};
/******************************************************************************************
Description : Add all revive actions to the wounded player object only
Syntax : [unit] call BAS_revive_fnc_addReviveActions;
Parameters : unit: Object
Return : None
*******************************************************************************************/
BAS_revive_fnc_addReviveActions =
{
private ["_unit", "_player"];
_unit = _this;
_player = player;
if ((_player == _unit) || ((BAS_revive_onlySameSideRevive) && (GET_SIDE(_player) != GET_SIDE(_unit)))) exitWith {}; // don't add action to yourself to revive yourself
/* Add actions to incapacitated player object */
_unit addAction ["<t color='#008000'>Stabilize</t>", "call BAS_revive_fnc_stabilizePlayer", nil, 9, true, true, "", "call BAS_revive_fnc_stabilizePlayerCheck", 2, false];
_unit addAction ["<t color='#008000'>Drag</t>", "call BAS_revive_fnc_dragPlayer", nil, 8, false, true, "", "call BAS_revive_fnc_dragPlayerCheck", 2, false];
/* Only add revive action to medic players */
if (_player getVariable "BAS_isMedic") then
{
_unit addAction ["<t color='#008000'>Revive</t>", "call BAS_revive_fnc_revivePlayer", nil, 10, true, true, "", "call BAS_revive_fnc_revivePlayerCheck", 2, false];
};
};
/******************************************************************************************
Description : Add action to execute wounded player
Syntax : [unit] call BAS_revive_fnc_addExecutionAction;
Parameters : unit: Object
Return : None
*******************************************************************************************/
BAS_revive_fnc_addExecutionAction =
{
private ["_unit", "_player"];
_unit = _this;
_player = player;
if ((_player == _unit) || ((BAS_revive_allowPlayerExecution) && (GET_SIDE(_player) == GET_SIDE(_unit)))) exitWith {}; // don't add action to yourself to revive yourself
/* Add action to incapacitated player object */
_unit addAction ["<t color='#FF0000'>Execute</t>", "call BAS_revive_fnc_executePlayer", nil, 7, true, true, "", "alive _this", 2, false];
};
/******************************************************************************************
Description : Check that all conditions are met to allow people to revive dead player
Syntax : [] call BAS_revive_fnc_revivePlayerCheck;
Parameters : Those from the addAction condition check
Return : None
*******************************************************************************************/
BAS_revive_fnc_revivePlayerCheck =
{
private ["_isRevived", "_isDragged", "_isIncapacitated"];
_isRevived = _target getVariable "BAS_isRevived";
_isDragged = _target getVariable "BAS_isDragged";
_isIncapacitated = _target getVariable "BAS_isIncapacitated";
if ((!alive _target) || (!alive _this) || (_isRevived) || (_isDragged) || (!_isIncapacitated) || (!("Medikit" in (items _this)))) exitWith { false };
true
};
/******************************************************************************************
Description : Revive an incapacitated unit
Syntax : [] call BAS_revive_fnc_revivePlayer;
Parameters : Those from the addAction execution
Return : None
*******************************************************************************************/
BAS_revive_fnc_revivePlayer =
{
private ["_victim", "_rescuer", "_EHanimDoneID"];
_victim = _this select 0;
_rescuer = _this select 1;
/* Avoid other action being triggered while reviving */
_victim setVariable ["BAS_isRevived", true, true];
/* Play revive animation */
SET_ANIM(_rescuer);
_EHanimDoneID = _rescuer addEventHandler ["AnimDone", BAS_revive_fnc_handleAnim];
if ((stance _rescuer) in ["STAND","CROUCH"]) then
{
_rescuer playMove "AinvPknlMstpSlayWrflDnon_medicOther";
}
else
{
_rescuer playMove "AinvPpneMstpSlayWrflDnon_medicOther";
};
waitUntil { ((GET_ANIM(_rescuer) != "") || (!alive _victim) || (!alive _rescuer) || (_rescuer getVariable "BAS_isIncapacitated")) };
_rescuer removeEventHandler ["AnimDone", _EHanimDoneID];
/* Revive Only when animation has finished */
if ((GET_ANIM(_rescuer) == "AinvPknlMstpSlayWrflDnon_medicOther") || (GET_ANIM(_rescuer) == "AinvPpneMstpSlayWrflDnon_medicOther")) then
{
_victim setVariable ["BAS_isIncapacitated", false, true];
SET_ANIM(_rescuer);
};
_victim setVariable ["BAS_isRevived", false, true];
};
/******************************************************************************************
Description : Check that all conditions are met to stabilize incapacitated player
Syntax : [] call BAS_revive_fnc_stabilizePlayerCheck;
Parameters : Those from the addAction condition check
Return : None
*******************************************************************************************/
BAS_revive_fnc_stabilizePlayerCheck =
{
private ["_isRevived", "_isDragged" , "_isStabilized", "_isIncapacitated"];
_isRevived = _target getVariable "BAS_isRevived";
_isDragged = _target getVariable "BAS_isDragged";
_isStabilized = _target getVariable "BAS_isStabilized";
_isIncapacitated = _target getVariable "BAS_isIncapacitated";
if ((!alive _target) || (!alive _this) || (_isRevived) || (_isDragged) || (_isStabilized) || (!_isIncapacitated) || ((!("Medikit" in (items _this))) && (!("FirstAidKit" in (items _this))))) exitWith { false };
true
};
/******************************************************************************************
Description : Stabilize an incapacitated player so he doesn't loose blood
Syntax : [] call BAS_revive_fnc_stabilizePlayer;
Parameters : Those from the addAction execution
Return : None
*******************************************************************************************/
BAS_revive_fnc_stabilizePlayer =
{
private ["_victim", "_rescuer", "_EHanimDoneID"];
_victim = _this select 0;
_rescuer = _this select 1;
/* Avoid other action being triggered while reviving */
_victim setVariable ["BAS_isRevived", true, true];
/* Play stabilize animation */
SET_ANIM(_rescuer);
_EHanimDoneID = _rescuer addEventHandler ["AnimDone", BAS_revive_fnc_handleAnim];
if ((stance _rescuer) in ["STAND","CROUCH"]) then
{
_rescuer playMove "AinvPknlMstpSlayWrflDnon_medicOther";
}
else
{
_rescuer playMove "AinvPpneMstpSlayWrflDnon_medicOther";
};
waitUntil { ((GET_ANIM(_rescuer) != "") || (!alive _victim) || (!alive _rescuer) || (_rescuer getVariable "BAS_isIncapacitated")) };
_rescuer removeEventHandler ["AnimDone", _EHanimDoneID];
if ((GET_ANIM(_rescuer) == "AinvPknlMstpSlayWrflDnon_medicOther") || (GET_ANIM(_rescuer) == "AinvPpneMstpSlayWrflDnon_medicOther")) then
{
_victim setVariable ["BAS_isStabilized", true, true];
SET_ANIM(_rescuer);
if (!("Medikit" in (items _rescuer))) then
{
_rescuer removeItem "FirstAidKit";
};
};
_victim setVariable ["BAS_isRevived", false, true];
};
/******************************************************************************************
Description : Check that all conditions are met to allow people to drag dead player
Syntax : [] call BAS_revive_fnc_dragPlayerCheck;
Parameters : Those from the addAction condition check
Return : None
*******************************************************************************************/
BAS_revive_fnc_dragPlayerCheck =
{
private ["_isRevived", "_isDragged", "_isIncapacitated"];
_isRevived = _target getVariable "BAS_isRevived";
_isDragged = _target getVariable "BAS_isDragged";
_isIncapacitated = _target getVariable "BAS_isIncapacitated";
if ((!alive _target) || (!alive _this) || (_isRevived) || (_isDragged) || (!_isIncapacitated)) exitWith { false };
true
};
/******************************************************************************************
Description : Drag an incapacitated unit
Syntax : [] call BAS_revive_fnc_dragPlayer;
Parameters : Those from the addAction execution
Return : None
*******************************************************************************************/
BAS_revive_fnc_dragPlayer =
{
private ["_victim", "_rescuer", "_releaseActionID"];
_victim = _this select 0;
_rescuer = _this select 1;
/* Avoid other action being triggered while dragging */
_victim setVariable ["BAS_isDragged", true, true];
/* Attach victim to rescuer */
_victim attachTo [_rescuer, [0,1,0]];
[_victim, 180] remoteExec ["BAS_revive_fnc_setDirFromRemote", _victim, false]; // execute setDir where victim is local
/* Play drag animation */
_rescuer playMove "AcinPknlMstpSrasWrflDnon";
[_victim, "AinjPpneMstpSnonWrflDnon"] call BAS_revive_fnc_switchMoveGlobal;
/* Add release action */
_releaseActionID = _rescuer addAction ["<t color='#228B22'>Release</t>", "call BAS_revive_fnc_releasePlayer", nil, 10, true, true, "", "alive _target", -1, false];
waitUntil { sleep 0.1; ((!alive _victim) || (!alive _rescuer) || (_rescuer getVariable "BAS_isIncapacitated") || (!(_victim getVariable "BAS_isDragged"))) };
if (_victim getVariable "BAS_isDragged") then
{
detach _victim;
if (alive _victim) then
{
[_victim, "AinjPpneMstpSnonWrflDnon"] call BAS_revive_fnc_switchMoveGlobal;
_victim setVariable ["BAS_isDragged", false, true];
};
if ((alive _rescuer) && (!(_rescuer getVariable "BAS_isIncapacitated"))) then
{
/* Play release animation */
_rescuer playMove "AmovPknlMstpSrasWrflDnon";
};
};
_rescuer removeAction _releaseActionID;
};
/******************************************************************************************
Description : Release dragged unit
Syntax : [] call BAS_revive_fnc_releasePlayer;
Parameters : Those from the addAction execution
Return : None
*******************************************************************************************/
BAS_revive_fnc_releasePlayer =
{
private ["_victim", "_rescuer"];
_rescuer = _this select 0;
/* Search for victim in attached object */
{
if (_x isKindOf "CAManBase") exitWith { _victim = _x }
} count attachedObjects _rescuer;
if (!isNull _victim) then
{
/* Detach victim */
detach _victim;
/* Clear dragged state */
_victim setVariable ["BAS_isDragged", false, true];
/* Play release animation */
_rescuer playMove "AmovPknlMstpSrasWrflDnon";
[_victim, "AinjPpneMstpSnonWrflDnon"] call BAS_revive_fnc_switchMoveGlobal;
};
};
/******************************************************************************************
Description : Execute an incapacitated player
Syntax : [] call BAS_revive_fnc_executePlayer;
Parameters : Those from the addAction execution
Return : None
*******************************************************************************************/
BAS_revive_fnc_executePlayer =
{
private ["_victim", "_executioner"];
_victim = _this select 0;
_executioner = _this select 1;
/* Play execution animation */
SET_ANIM(_executioner);
_EHanimDoneID = _executioner addEventHandler ["AnimDone", BAS_revive_fnc_handleAnim];
_executioner playMove "Acts_Executioner_Kill";
waitUntil { ((GET_ANIM(_executioner) != "") || (!alive _victim) || (!alive _executioner) || (_executioner getVariable "BAS_isIncapacitated")) };
_executioner removeEventHandler ["AnimDone", _EHanimDoneID];
if (GET_ANIM(_executioner) == "Acts_Executioner_Kill") then
{
_victim setDamage 1;
};
};
/******************************************************************************************
Description : Handle death of the player
Syntax : unit call BAS_revive_fnc_handleDeath;
Parameters : None
Return : None
*******************************************************************************************/
BAS_revive_fnc_handleDeath =
{
private _corpse = _this select 0;
/* Remove captive status */
_unit setCaptive false;
/* Delete all variables */
_corpse setVariable ["BAS_isMedic", nil, true];
_corpse setVariable ["BAS_isEngineer", nil, true];
_corpse setVariable ["BAS_isIncapacitated", nil, true];
_corpse setVariable ["BAS_isRevived", nil, true];
_corpse setVariable ["BAS_isStabilized", nil, true];
_corpse setVariable ["BAS_isDragged", nil, true];
_corpse setVariable ["BAS_animDone", nil, true];
_corpse setVariable ["BAS_playerSide", nil, true];
_corpse setVariable ["BAS_revive_initDone", nil, true];
/* Remove all event handlers */
_corpse removeAllEventHandlers "HandleDamage";
_corpse removeAllEventHandlers "Killed";
_corpse removeAllEventHandlers "AnimDone";
/* Remove all actions */
_corpse remoteExec ["removeAllActions", [0,-2] select isDedicated, false];
};
/******************************************************************************************
Description : Add "hide body" action on a corpse
Syntax : unit call BAS_revive_fnc_addHideBodyAction;
Parameters : Unit: Object
Return : None
*******************************************************************************************/
BAS_revive_fnc_addHideBodyAction =
{
_this addAction ["<t color='#FF8C00'>Hide Body</t>", "call BAS_revive_fnc_hideBody", nil, 0, false, true, "", "alive _this", 2, false];
};
/******************************************************************************************
Description : Slowly burry a dead body
Syntax : [] call BAS_revive_fnc_hideBody;
Parameters : None
Return : None
*******************************************************************************************/
BAS_revive_fnc_hideBody =
{
private ["_corpse", "_unit"];
_corpse = _this select 0;
_unit = _this select 1;
/* Burry the dead body */
_unit action ["hideBody", _corpse];
/* Wait until corpse is effectively deleted */
waitUntil {sleep 0.1; isNull _corpse };
/* Remove add hide body action from JIP queue */
remoteExec ["", _corpse];
/* Remove corpse assigned actions from all clients */
_corpse remoteExec ["removeAllActions", [0,-2] select isDedicated, false];
};
/******************************************************************************************
Description : Broadcast switchMove command over the network
Syntax : [unit, animation] call BAS_revive_fnc_switchMoveGlobal;
Parameters : Unit: Object
Animation: String
Return : None
*******************************************************************************************/
BAS_revive_fnc_switchMoveGlobal =
{
[_this select 0, _this select 1] remoteExec ["switchMove", [0,-2] select isDedicated, false];
};
/******************************************************************************************
Description : Set direction of given unit where it is local
Syntax : [unit, direction] call BAS_revive_fnc_setDirFromRemote;
Parameters : Unit: Object
Direction: Number (0-360)
Return : None
*******************************************************************************************/
BAS_revive_fnc_setDirFromRemote =
{
(_this select 0) setDir (_this select 1);
};
/******************************************************************************************
Description : Test if given projectile is whitelisted (revive allowed)
Syntax : projectile call BAS_revive_fnc_isProjectileNonLethal;
Parameters : Projectile: Object
Return : Boolean
*******************************************************************************************/
BAS_revive_fnc_isProjectileNonLethal =
{
private _return = false;
{
if (_this isKindOf [_x, configFile >> "CfgAmmo"]) exitWith { _return = true }
} count BAS_revive_projectileWhiteList;
_return
};
STEF 5 : inside BAS_revive folder, make BAS_revive_initialization.sqf file and past inside :
​
​
/******************************************************************************************
* This work is licensed under the APL-SA. Copyright © 2016 basstard @ BI Forums *
******************************************************************************************
Author : basstard
Date : 19/08/2016 (jj/mm/aaaa)
Version : 0.1
Description : Initialization of basstard's revive context
Usage : This script is called from init.sqf
Syntax : call compileFinal preprocessFileLineNumbers "BAS_revive\BAS_revive_initialization.sqf";
Parameters : None
*/
//#define BAS_DEBUG
/* Global vars that can be tweaked */
BAS_revive_bleedOut = 90; // time in seconds until you die after being incapacitated, bleed out can't be disabled (BAS_revive_bleedOut = 0 -> insta death)
#ifdef BAS_DEBUG
BAS_revive_onlySameSideRevive = false; // don't tweak this one, use next !
#else
BAS_revive_onlySameSideRevive = true; // true: you can revive players from your side only, false: you can revive whoever is incapacitated
#endif
BAS_revive_allowPlayerExecution = true; // when player is incapacitated, he can be executed by people which are not from his side
BAS_revive_projectileWhiteList = // list of projectiles that will cause incapacitated state (if the player is severely wounded by a projectile not in this list he will die)
[
"B_556x45_Ball",
"B_65x39_Caseless",
"B_762x51_Ball",
"B_762x54_Ball",
"B_9x21_Ball",
"B_45ACP_Ball",
"B_762x39_Ball_F",
"B_93x64_Ball",
"B_127x54_Ball",
"B_580x42_Ball_F",
"B_545x39_Ball_F"
];
/* Compile functions linked to revive functionnalities */
call compile preprocessFile "addons\BAS_revive\BAS_revive_functions.sqf";
/* Initialize player on client only */
if (!isDedicated) then
{
[] spawn BAS_revive_fnc_playerInit;
};
STEF 6 : inside BAS_revive folder, make BAS_revive_macros.sqf file and past inside :
​
​
​
/******************************************************************************************
* This work is licensed under the APL-SA. Copyright © 2016 basstard @ BI Forums *
******************************************************************************************
Author : basstard
Date : 03/09/2016 (jj/mm/aaaa)
Version : 0.1
Description : Macro collection for basstard's revive functionnalities
Usage : N/A
Syntax : N/A
Parameters : None
*/
/* Get the last played animation */
#define GET_ANIM(UNIT) (UNIT getVariable ["BAS_animDone", ""])
/* Reset the last played animation */
#define SET_ANIM(UNIT) (UNIT SetVariable ["BAS_animDone", "", true])
/* Get cached player side */
#define GET_SIDE(UNIT) (UNIT getVariable "BAS_playerSide")
/* Cache player side */
#define SET_SIDE(UNIT) (UNIT setVariable ["BAS_playerSide", side UNIT, true])
/* Damage modifier for overall damage selection */
#define OVERALL_DAMAGE_MODIFIER 0.5 // use if you want overall damage not increasing too much (because legs and arms damages also add overall damages), set to 1 to have the default Arma behavior
​
​
STEP 7 : go back to your map, save and export in multiplayer
​